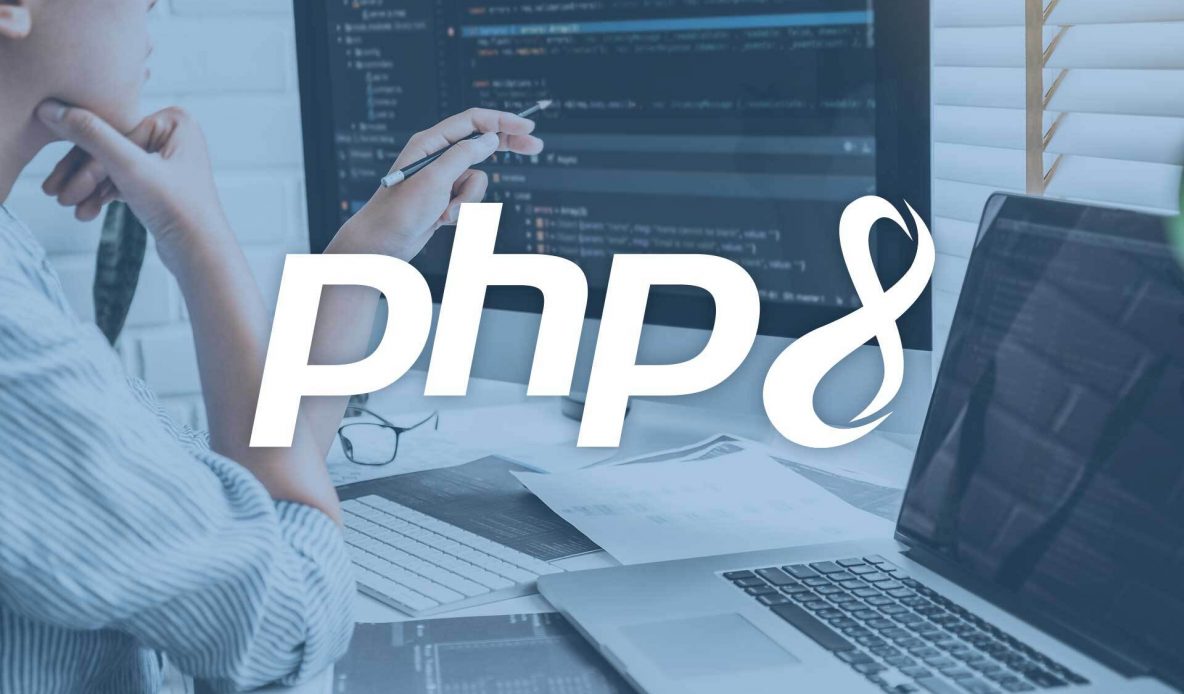
7 New Features We’re Most Looking Forward to in PHP 8
With the upcoming release of PHP 8 on November 26th comes a litany of new features for the language. We sat down with two of our back-end developers, Brandon Fenning and Charles Punchatz, to find out which of these features they’re most looking forward to using when they drop later this year. Here is what they had to say:
Features
1. Improved Typehinting
Charles: Originally a loosely typed language, typehinting was introduced in PHP 5 and improved and expanded in PHP 7. With this latest release, PHP will continue to improve hinting in several ways:
- It introduces union types, allowing developers to specify multiple arguments or return types
- Adds a
$1
type, allowing developers to explicitly specify that an argument or return type can return any type - Adds the
static
return type - Adds the
Stringable
interface - Adds type annotations in internal functions
- Standardizes type errors
- Adds stricter type checks to arithmetic operators
- Validation for abstract trait methods
Typehinting has plenty of advantages—code becomes self documenting, easier to understand, and allows developers to catch bugs earlier—so we’re always happy to see improvements.
Of all of this, I’m most looking forward to implementing union types. Here at Lform we work with WordPress on many projects, and while WordPress has many advantages for our clients, it can create a headache for our developers due in part to its inconsistencies in how it returns or expects data. With the introduction of union types, the code we write on top of WordPress can account for these inconsistencies, improving its readability and maintainability.
Brandon: To add to what Charles said, typing is a key feature of modern programming languages as it allows errors to be caught at compile time. While no-typing or weak typing is often seen as easier to use by beginners, in the long run, it leads to more bugs that would have been caught by the PHP runtime compiler. Continuing its journey towards stronger typing, PHP 8 has built upon the typing features it introduced in version 7 with some new additions.
- Union Type: Previously relegated to PHP DocBlocks, PHP 8 now has ‘union types’ which allow for multiple types to be specified in properties, arguments, and return types. Unlike DocBlocks, these are checked at compile time.
- Static Return Type: While a class method could return
self
orparent
, they could not returnstatic
which introduced its own inheritance issues with classes & patterns likereturn $this
. - Mixed return types: While mixed return types go against the general philosophy behind strong typing, the new
mixed
type is a suggestion that if we wish to be ‘improper’, we might as well be explicitly improper so there’s no confusion on the part of either the coder or the compiler about the code’s intent.
2. Named Parameters
Charles: Named parameters have existed for a long time in many other popular languages such as C#, Kotlin, Python, R, Ruby, Sass, and Swift. Named parameters allow developers to pass data into a function, according to their parameter name instead of their order, making parameters order-independent and allowing developers to skip default values arbitrarily.
While there are many arguments as to why this may not be necessary, named parameters will allow us to write less code, especially when dealing with legacy systems. For example, we have a function built into both our WordPress and Ada systems to truncate text:
function synopsis(string $string, int $maxLength = 100, string $ending = '…', bool $respectBreaks = true): string;
In using this function on multiple projects, I’ve found that the $respectBreaks
parameter is changed much more frequently than, for example, the $ending
parameter—it’s a rare occurrence where truncated text is not followed by an ellipsis while it is more frequent that line breaks are stripped.
This results in the following:
synopsis($someText, 100, ‘…’, false);
While we could simply change the function signature, that would break many (if not all) instances where this function is used across two CMS systems.
Instead, named parameters allow us to simply specify the parameter name we want to pass and skip the rest:
synopsis($someText, respectBreaks: true);
Not only will this simplify our code when dealing with some of our own legacy systems, but also when dealing with functions we cannot control, such as those built into PHP’s core or introduced by the frameworks and systems we often use.
3. First Class Citizen Attributes
Brandon: PHP has finally added compiler-recognized meta attributes. While some frameworks parse PHP DocBlocks, this feature finally makes adding annotations/attributes an intrinsic part of PHP. There’s a lot to read up about attributes, but the biggest thing is that since attributes are classes, unlike DocBlocks, this means you can create your own attributes and apply them to your code-base depending on your needs. This data can also be read by reflection classes.
4. Object class resolution via ::class
Brandon: Adding to the system’s consistency, objects can now access their class namespace via
$object::class
just like classes can already do via NiceClass::class
.
Charles: While what Brandon brings up isn’t a world-breaking change, it keeps things consistent and passed unanimously as a result. PHP 5.5 first introduced class name resolution, which allowed us to get the fully-qualified name of a class. This is the perfection continuation.
5. Nullsafe Operator
Charles: The null coalescing operator introduced in PHP 7, and null coalescing assignment, introduced in PHP 7.4, have saved us a lot of time by eliminating the need to write lengthy, repetitive code to provide default values for unassigned variables. It does have one glaring flaw, though. It does not work on chained object properties or method calls. Until now, we still had to write lengthy code to avoid errors for these situations. Considering the following example, very common when we use WordPress in conjunction with TypeRocket:
$var = $post->meta->section->title;
As is, the code can fail if $post->meta
or $post->meta->section
return null
, requiring us to write something like the following every time we grab meta data for a post:
$var = $post->meta ? ($post->meta->section ? $post->meta->section->title : null) : null;
With the nullsafe operator, the above code can be simplified to:
$var = $post->meta?->section?->title;
Resulting in much simpler, cleaner, and easier to read code.
Brandon: The simple truth is that the nullsafe operator allows for less code by simplifying common flow-control strategies when chaining methods hands down.
6. New String Functions
Charles: Long overdue are the inclusion of three new built in functions:
While these types of functions are present in other languages, such as JavaScript, they were only achievable in PHP through a combination of other functions such as strpos()
, substr()
, and strlen()
, among others. This minimizes the overhead of multiple function calls and adds an oft-used feature to the core of the language.
Brandon: In addition to what Charles mentioned, I think the simple task of finding out if one string is inside another string is a common stumbling block for new coders in PHP. Generally, this is accomplished via the
strpos()
function, which introduces the possibility of overlooking its mixed return type of 0
vs false
if the match is located at the start of the string. Now we can accomplish this with three new functions, as Charles mentioned above. The result is more readable code which is a win-win for everyone.
7. Switch up Matching
Brandon: The new
match
expression allows for slimmer implementations that previously would have used the more verbose switch
expression:
/**
* PHP 7.x Implementation:
*/
switch ($value) {
case 'nice':
case 'other_nice':
$result = doOtherNice();
break;
case 'swell':
$result = doSwellStuff();
break;
}
/**
* PHP 8.x Implementation:
*/
$result = match ($value) {
'nice', 'other_nice' => doOtherNice(),
'swell' => doSwellStuff(),
}
In Conclusion
With the arrival of PHP 8 comes a multitude of features and enhancements that will help developers write cleaner, more succinct, self-documenting code all while improving performance. While we are most excited to be able to implement the above, we’re looking forward to getting our hands dirty with the next big iteration of the language.